This application only needed when on the same server you have different folders that each of them requires different account.
Platform : C# 2010
Operating System : Windows
2000 /
XP /
2003 /
Vista /
win7 (32bit)
Requirements : v4 .NET framework
Filesize : 53kb
Download
RunasX - runs an application under specific domain\user, user can pass the details (domain, username, password) via commandline. Solves the problem on Windows Home Editions where user cant store the credentials to credential manager, using runas /savecred.

similar
run the program with different user
https://www.nirsoft.net/utils/advanced_run.html
Windows 7 home premium does not have the feature join a domain network, via system properties or by shift + right click an icon 'run as different user'. While you can't join a domain there are ways you can connect to a domain for running applications if you have a domain account. You can use the runas /netonly command:
extended solution to accept LM, NTLM, and NTLMv2 session security
Windows - Credential Manager
Windows 7: Elevated Program Shortcut
finally the solution for Windows 7 Home Edition is CreateProcessWithLogonW API :
The network folder specified is currently mapped using a different user name and password.To connect using a different user name and password, first disconnect any existing mappings to this network share.
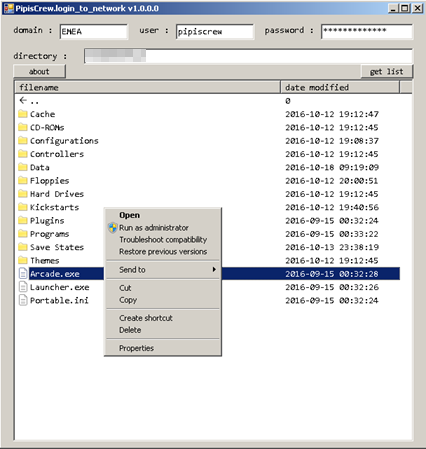
Platform : C# 2010
Operating System : Windows





Requirements : v4 .NET framework
Filesize : 53kb
Download
similar
run the program with different user
https://www.nirsoft.net/utils/advanced_run.html
Windows 7 home premium does not have the feature join a domain network, via system properties or by shift + right click an icon 'run as different user'. While you can't join a domain there are ways you can connect to a domain for running applications if you have a domain account. You can use the runas /netonly command:
JavaScript:
//src https://superuser.com/a/917346
runas /netonly /user:mydomain\username "pathToFile/file.exe"
ex. runas /netonly /user:dataxstream\username "C:\Program Files (x86)\Microsoft SQL Server\...\Ssms.exe"
extended solution to accept LM, NTLM, and NTLMv2 session security
JavaScript:
//src - https://superuser.com/a/1096731
Access HKEY_LOCAL_MACHINE\SYSTEM\CurrentControlSet\Control\Lsa
Create a new DWORD (32bit) entry called LmCompatibilityLevel
Modify the entry and set the value to 1
Reboot required.
//LmCompatibilityLevel - https://library.netapp.com/ecmdocs/ECMP1401220/html/GUID-55B2F618-A90A-44FC-BA6E-92098E94D79A.html
Windows - Credential Manager
Windows 7: Elevated Program Shortcut
JavaScript:
//src - https://superuser.com/a/368776 -- is not available on Windows 7 Home
//you will prompted for the password, then will be saved to Cpanel > Credenrials Manager
runas /savecred /user:domain\user "C:\Windows\notepad.exe"
finally the solution for Windows 7 Home Edition is CreateProcessWithLogonW API :
JavaScript:
//sample on C#
//
//src - https://gist.github.com/adamdriscoll/4effdf9d29228d1e9f1184bfd92d744b
//ref - https://stackoverflow.com/a/15628228/1026
//ref - https://serverfault.com/a/999680
using System;
using System.ComponentModel;
using System.Runtime.ConstrainedExecution;
using System.Runtime.InteropServices;
using System.Security;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
CreateProcessAsUser(@"C:\Program Files (x86)\SSMS\Common7\IDE\Ssms.exe", "", "YouRusername", "YouRdomainName", "YouRpassword");
}
public void CreateProcessAsUser(string filepath, string arguments, string username, string domainName, string password)
{
var si = new STARTUPINFO();
var pi = new PROCESS_INFORMATION();
if (!CreateProcessWithLogonW(username, domainName, password,
LogonFlags.LOGON_NETCREDENTIALS_ONLY, null, filepath + " " + arguments,
CreationFlags.CREATE_DEFAULT_ERROR_MODE, 0, null, ref si, out pi))
{
throw new Win32Exception();
}
//WaitForSingleObject(pi.hProcess, 0xffffffff);
//CloseHandle(pi.hProcess);
//CloseHandle(pi.hThread);
Application.Exit();
}
[DllImport("kernel32.dll", SetLastError = true)]
static extern UInt32 WaitForSingleObject(IntPtr hHandle, UInt32 dwMilliseconds);
[DllImport("advapi32.dll", SetLastError = true, CharSet = CharSet.Unicode)]
private static extern bool CreateProcessWithLogonW(
String userName,
String domain,
String password,
LogonFlags logonFlags,
String applicationName,
String commandLine,
CreationFlags creationFlags,
UInt32 environment,
String currentDirectory,
ref STARTUPINFO startupInfo,
out PROCESS_INFORMATION processInformation);
[DllImport("kernel32.dll", SetLastError = true)]
[ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)]
[SuppressUnmanagedCodeSecurity]
[return: MarshalAs(UnmanagedType.Bool)]
static extern bool CloseHandle(IntPtr hObject);
[Flags]
enum CreationFlags
{
CREATE_SUSPENDED = 0x00000004,
CREATE_NEW_CONSOLE = 0x00000010,
CREATE_NEW_PROCESS_GROUP = 0x00000200,
CREATE_UNICODE_ENVIRONMENT = 0x00000400,
CREATE_SEPARATE_WOW_VDM = 0x00000800,
CREATE_DEFAULT_ERROR_MODE = 0x04000000,
}
[Flags]
enum LogonFlags
{
LOGON_WITH_PROFILE = 0x00000001,
LOGON_NETCREDENTIALS_ONLY = 0x00000002
}
[StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)]
private struct STARTUPINFO
{
public Int32 cb;
public string lpReserved;
public string lpDesktop;
public string lpTitle;
public Int32 dwX;
public Int32 dwY;
public Int32 dwXSize;
public Int32 dwYSize;
public Int32 dwXCountChars;
public Int32 dwYCountChars;
public Int32 dwFillAttribute;
public Int32 dwFlags;
public Int16 wShowWindow;
public Int16 cbReserved2;
public IntPtr lpReserved2;
public IntPtr hStdInput;
public IntPtr hStdOutput;
public IntPtr hStdError;
}
[StructLayout(LayoutKind.Sequential)]
private struct PROCESS_INFORMATION
{
public IntPtr hProcess;
public IntPtr hThread;
public int dwProcessId;
public int dwThreadId;
}
}
}